[Spring/Thymeleaf] 타임리프로 팝업창 띄워보기 (feat. div 수직 중앙 정렬)
siso 프로젝트를 진행하기 위해 관리자는 웹으로 접근하도록 하기 위해 타임리프를 통해 html을 짜야 했다. 근데 1학년 때 html을 접해보고 그 뒤로 아예 접해보지 못해 너무 어려웠다.. 오늘은 타임
9hyuk9.tistory.com
오늘 해볼 것은 자바스크립트를 사용하여
부모 창에서 자식 창을 띄우고 자식 창 데이터를 부모 창으로 보내보자!
먼저 완성된 모습은 다음과 같다.
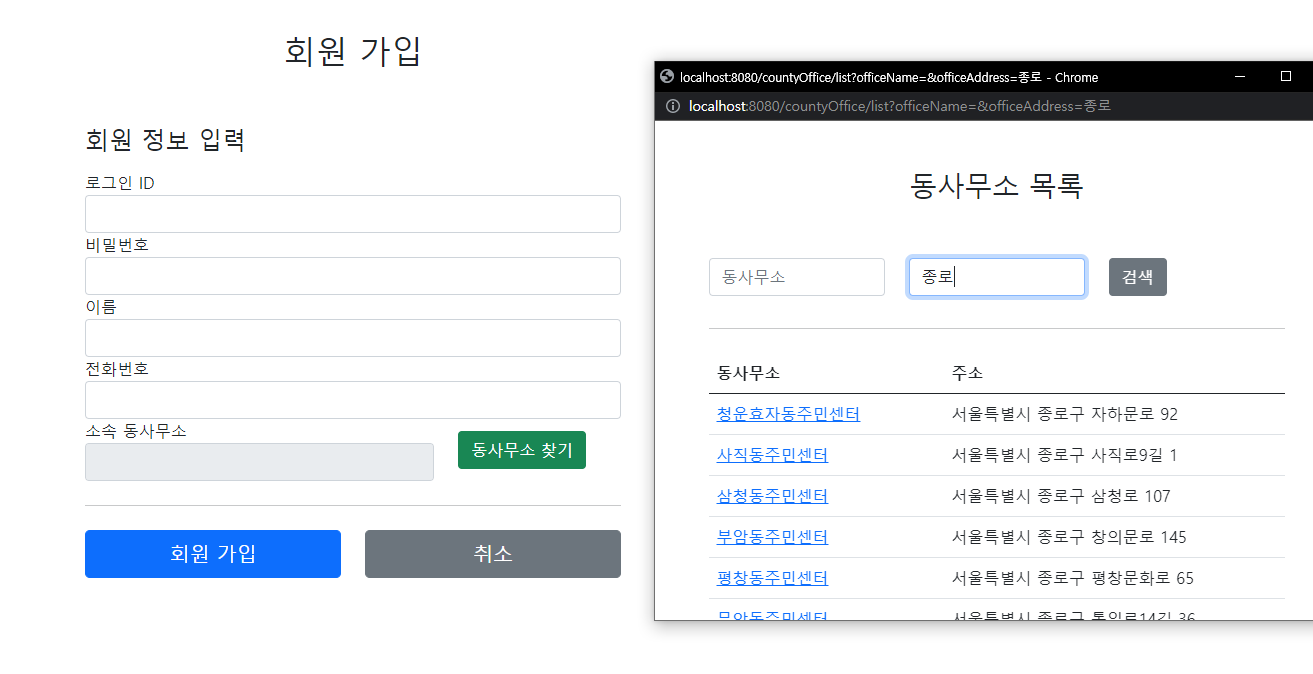
동사무소 찾기를 누르면 자식 창을 띄운 후 동사무소를 선택하면
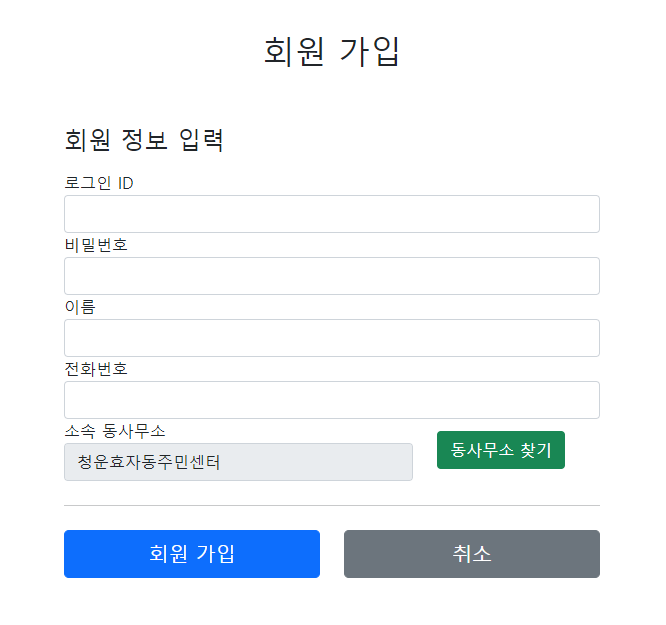
이렇게 기입이 된다.
그러면 일단 어떻게 하는지 알아보자!
[부모 창] 자식 창을 띄우는 JS 함수
window.onload = function(){
document.getElementById("newwin").onclick = function(){
window.open("/countyOffice/list","","width=700px,height=500px,top=200px,left=200px;");
}
};
newwin이라는 id를 가진 버튼이 클릭되었을 경우 위의 JS 함수가 실행된다.
window.open(주소, 사이즈);
[부모 창] newwin ID를 가진 버튼으로 JS 함수 호출하자!
<div class="col-md-4">
<button id="newwin" class="w-80 btn btn-success" type="button">동사무소 찾기
</button>
</div>
자바 스크립트에서 설정한 newwin을 버튼 ID에 주면 된다!
그래서 클릭 이벤트가 발생할 때마다 자바 스크립트 펑션이 실행된다.
[자식 창] 자식 창에서 데이터를 부모창에 보내보자!
일단 나는 모델 객체를 자식창에서 가져오는데 이때 객체로 부모 창으로 보내고 싶었지만 삽질 후에도 되지 않아 동사무소 이름과 동사무소의 DB ID만 보냈다.
보내는 방법은 다음과 같다.
동사무소 객체들에게 href="#" 값을 주어 클릭이 가능하게 하고 클릭 시에 JS 함수가 실행되도록 하였다.
JS로 부모 창에 데이터를 보내는 함수
<script type="text/javascript">
function sendCountyOffice(id, name){
window.opener.setCountyOffice(id, name);
window.close();
}
</script>
코드를 읽는 그대로 ID와 동사무소 이름을 가져와 그대로 부모 창의 함수로 보냈다.
그리고 window.close();를 통하여 자식 창 꺼주기!
타임리프에서 클릭 시 JS 함수 호출
<tr th:each="countyOffice : ${countyOfficeList}">
<td><a href="#" th:onclick="sendCountyOffice([[${countyOffice.id}]], [[${countyOffice.officeName}]])"
th:text="${countyOffice.officeName}">동사무소</a>
</td>
<td><a th:text="${countyOffice.officeAddress}">주소</a></td>
</tr>
위 코드는 반복문을 돌려 동사무소 정보를 보내주는데
우리는 동사무소를 클릭 시에만 JS 코드를 호출할 것이니 th:onclick 시 sendCountyOffice를 호출하고
이때 매개변수는 [[${}]] 형식으로 줘야 한다!
그리고 href="#"은 위에서 말했듯이 별다른 동작은 안 하고 클릭을 가능하게 해 준다.
[부모 창] 자식 창에서 보내진 데이터를 필드에 채워보자!
JS에서 필드 값을 채워주는 함수
function setCountyOffice(id, name){
document.getElementById('countyOfficeId').value = id;
document.getElementById('countyOfficeName').value = name;
}
자식 창에서 이어받은 데이터를 value 값에 대입하여 필드를 채워준다.
필드값을 채울 부분
<div class="col">
<label for="name">소속 동사무소</label>
<input type="text" id="countyOfficeName" class="form-control" disabled>
<div class="field-error" th:errors="*{countyOfficeId}"/>
</div>
<div class="col" style="display: none;">
<label for="name">소속 동사무소 ID</label>
<input type="text" id="countyOfficeId" th:field="*{countyOfficeId}" class="form-control"
th:errorclass="field-error">
</div>

나의 경우 소속 동사무소 이름을 입력받는 곳이 위와 같이 하나로 보이지만
ID를 입력 받는 창을 style="display: none;" 속성을 주어 안 보이게 했다.
따라서 JS 함수가 실행되면 소속 동사무소 이름이 찰 것이고, 눈에는 안 보이지만 ID도 찰 것이다!
근데 여기서 진짜 주의점은 소속 동사무소 ID도 건들지 못하도록 input에 disabled를 주었는데 눈에는 값이 채워져 있어도 NULL 값으로 처리가 되었다!
그래서 꼭 disabled를 빼야 한다.
disabled 속성이 되어있으면 필드 값이 안 채워지는 것 같다.
(이거로 2시간 날렸습니다..)
전체 코드
부모 창
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="utf-8">
<link th:href="@{/css/bootstrap.min.css}"
href="../css/bootstrap.min.css" rel="stylesheet">
<style>
...
</style>
<th:block>
<script th:inline="javascript">
function setCountyOffice(id, name){
document.getElementById('countyOfficeId').value = id;
document.getElementById('countyOfficeName').value = name;
}
window.onload = function(){
document.getElementById("newwin").onclick = function(){
window.open("/countyOffice/list","","width=700px,height=600px,top=200px,left=200px;");
}
};
</script>
</th:block>
</head>
<body>
<div class="container">
<div class="py-5 text-center">
<h2>회원 가입</h2>
</div>
<h4 class="mb-3">회원 정보 입력</h4>
<form action="" th:action th:object="${admin}" method="post">
...
<div class="row align-items-center">
<div class="col">
<label for="name">소속 동사무소</label>
<input type="text" id="countyOfficeName" class="form-control" disabled>
<div class="field-error" th:errors="*{countyOfficeId}"/>
</div>
<div class="col" style="display: none;">
<label for="name">소속 동사무소 ID</label>
<input type="text" id="countyOfficeId" th:field="*{countyOfficeId}" class="form-control"
th:errorclass="field-error">
</div>
<div class="col-md-4">
<button id="newwin" class="w-80 btn btn-success" type="button">동사무소 찾기
</button>
</div>
</div>
...
</div>
</form>
</div>
</body>
</html>
자식 창
<!DOCTYPE html>
<html lang="ko" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="utf-8">
<link th:href="@{/css/bootstrap.min.css}"
href="css/bootstrap.min.css" rel="stylesheet">
<script type="text/javascript">
function sendCountyOffice(id, name){
window.opener.setCountyOffice(id, name);
window.close();
}
</script>
</head>
<body>
<div class="container" style="max-width: 600px">
<div class="py-5 text-center">
<h2>동사무소 목록</h2>
</div>
...
<hr class="my-4">
<div>
<table class="table">
<thead>
<tr>
<th>동사무소</th>
<th>주소</th>
</tr>
</thead>
<tbody>
<tr th:each="countyOffice : ${countyOfficeList}">
<td><a href="#" th:onclick="sendCountyOffice([[${countyOffice.id}]], [[${countyOffice.officeName}]])"
th:text="${countyOffice.officeName}">동사무소</a>
</td>
<td><a th:text="${countyOffice.officeAddress}">주소</a></td>
</tr>
</tbody>
</table>
</div>
</div>
</body>
</html>